Adding a Date Range Picker to SvelteKit
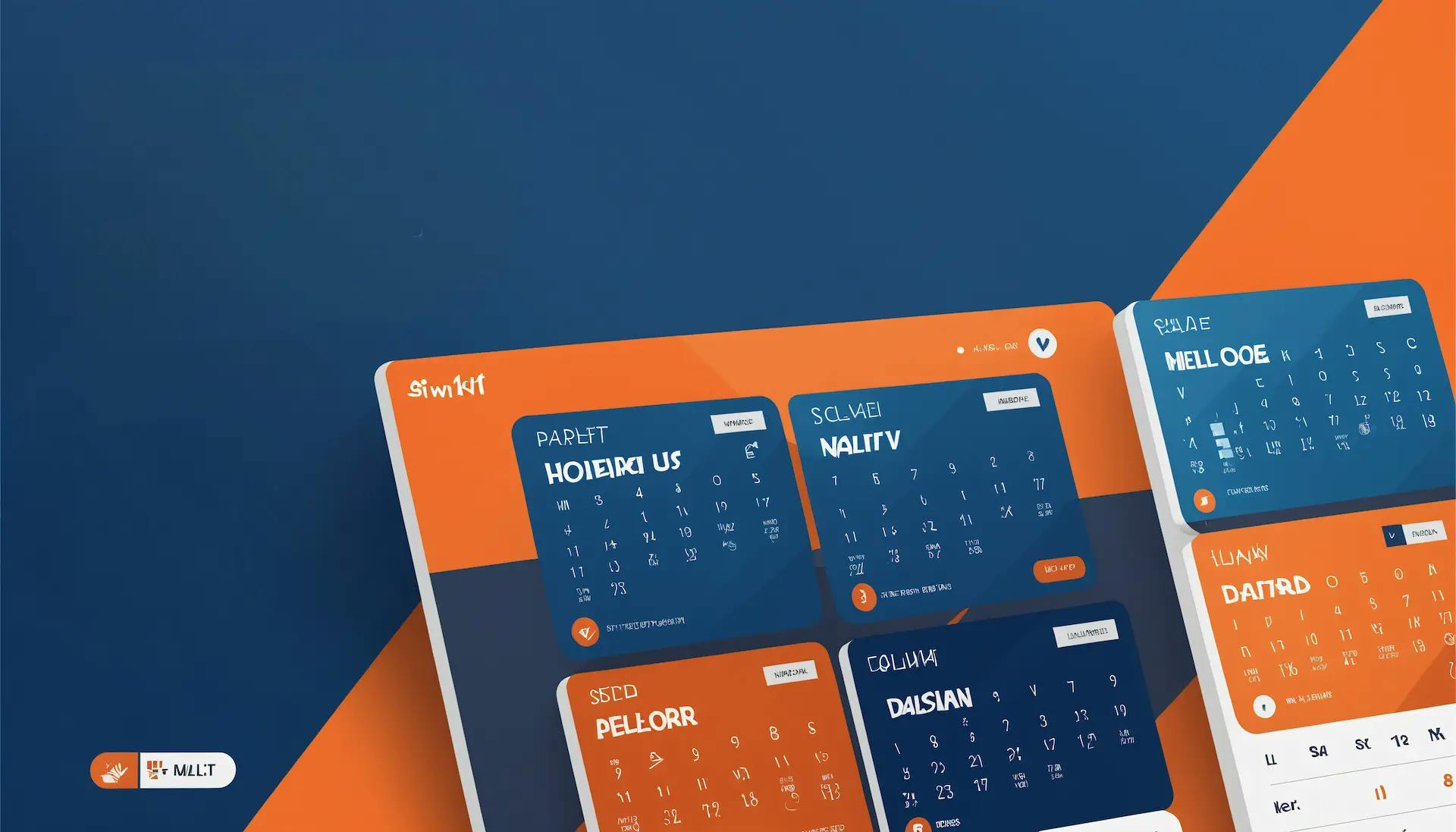
Check out the demo sveltekit-dateRangePicker.
If you haven't worked with any existing projects, you can check my blog and video tutorial about how to install a SvelteKit project efficiently. Follow the detailed installation guide for SvelteKit here: SvelteKit Installation Guide
Install Date Range Picker Install moment.js
# install daterangepicker
npm install daterangepicker
# install moment
npm install moment
Note: Since
moment.js
is no longer actively developed, consider using alternatives likeDay.js
ordate-fns
in future projects.
import { onMount } from "svelte";
import daterangepicker from "daterangepicker";
let dateContainerID = "#dateRange";
let DateSelected = "";
onMount(() => {
const options = {
startDate: moment().subtract(6, "days"),
endDate: moment(),
opens: "right",
alwaysShowCalendars: true,
ranges: {
"Last 7 Days": [moment().subtract(6, "days"), moment()],
"Last 30 Days": [moment().subtract(29, "days"), moment()],
"This Month": [moment().startOf("month"), moment().endOf("month")],
"Last Month": [
moment().subtract(1, "month").startOf("month"),
moment().subtract(1, "month").endOf("month"),
],
},
};
new daterangepicker(dateContainerID, options, (start, end) => {
DateSelected =
start.format("YYYY-MM-DD") + " to " + end.format("YYYY-MM-DD");
console.log("New date range selected: " + DateSelected);
});
});
<main>
<div class="m-auto text-center my-5">
<input
type="text"
id="dateRange"
value=""
class="w-1/2 bg-orange-700 text-white font-bold"
/>
</div>
</main>
npm run dev -- --host
This command runs the SvelteKit app and makes it accessible on your network.
Due to module format issues, moment.js
needs to be included differently:
moment
Importimport { onMount } from "svelte";
import daterangepicker from "daterangepicker";
<!-- Moment.js and DateRangePicker CSS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.4/moment.min.js"></script>
<link
rel="stylesheet"
type="text/css"
href="https://cdn.jsdelivr.net/npm/daterangepicker/daterangepicker.css"
/>
DONE