Blender Naming Conventions to Write Clean and Consistent Scripts
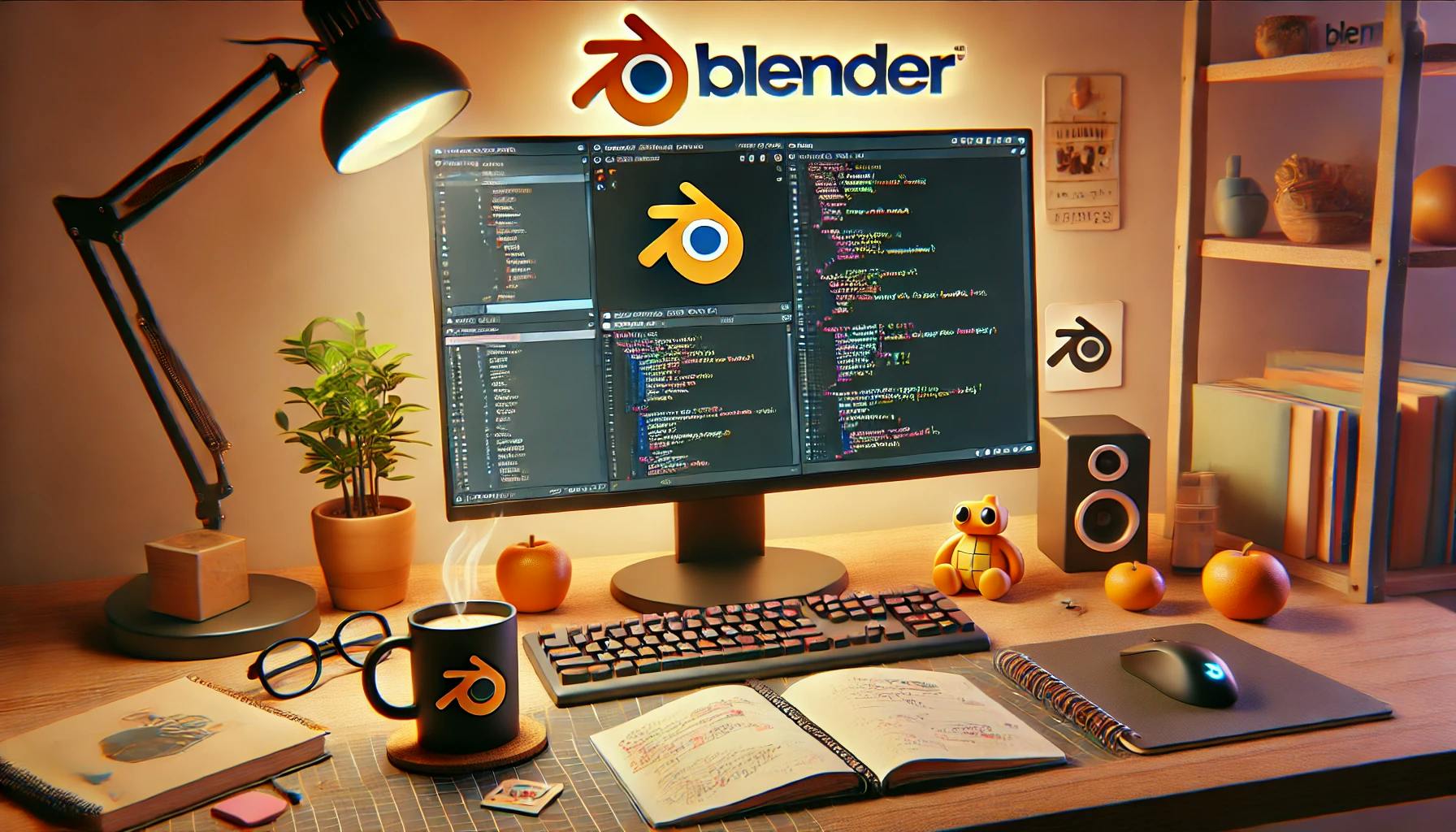
Blender scripting requires following specific naming conventions to maintain code clarity and consistency. Here’s a guide to help you keep your code in line with Blender’s best practices.
_
to separate words. [SPACE OR SCREEN NAME in UPPERCASE]
+ _
+ [PANEL(PT) or OPERATOR(OT) in UPPERCASE]
+ _
+ [Your Operator or Panel name in CamelCase]
. snake_case
(lowercase words separated by underscores).def record_macro_action(scene):
pass
camelCase
or PascalCase
for functions. These styles are typically reserved for class names. execute(self, context)
: Contains the main logic for the operator. It is called when the operator runs. invoke(self, context, event)
: Used when the operator is called interactively (e.g., from a button click). modal(self, context, event)
: Used for operators that run in a continuous loop, typically for interactive tools. draw(self, context)
: Defines how an operator's properties are displayed in the UI. Example of an operator class:
class SimpleOperator(bpy.types.Operator):
bl_idname = "object.simple_operator"
bl_label = "Simple Operator"
def execute(self, context):
# Logic for the operator
return {'FINISHED'}
draw
): Fixed Namesbpy.types.Panel
), the draw
method is fixed and must be named draw
. This method is responsible for adding UI elements (buttons, labels, etc.) to the panel.class VIEW3D_PT_MacroRecorder(bpy.types.Panel):
bl_label = "Macro Recorder"
bl_idname = "VIEW3D_PT_macro_recorder"
bl_space_type = 'VIEW_3D'
bl_region_type = 'UI'
bl_category = 'Macro Recorder'
def draw(self, context):
layout = self.layout
layout.operator("wm.record_macro", text="Start/Stop Recording")
def _get_selected_object(context):
return context.active_object
def export_to_text_block(context):
pass
depsgraph_update_post
), use descriptive names to indicate their role:def record_macro(scene):
pass