Delaying Function Execution in SvelteKit Applications on Both Client and Server Sides
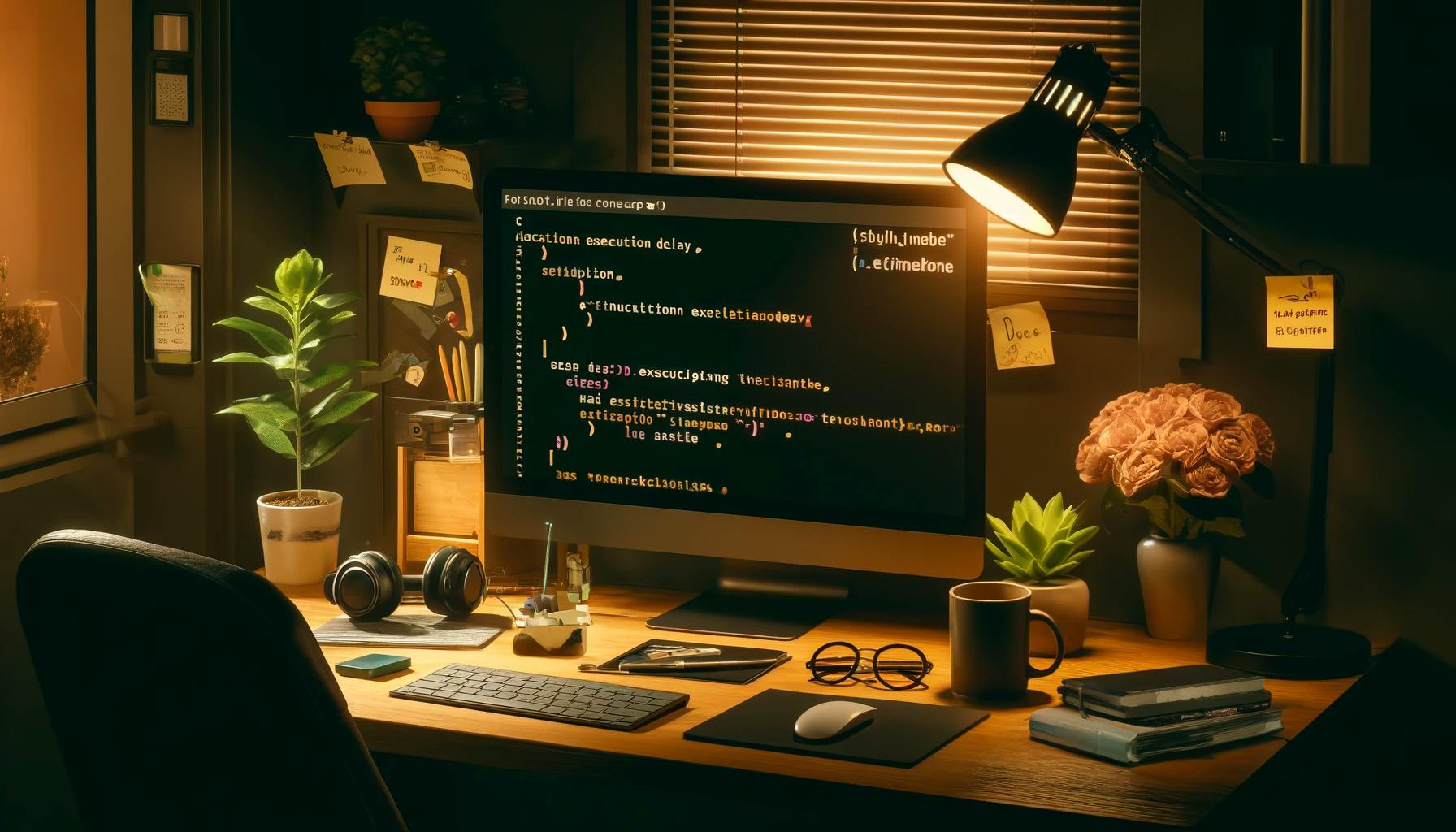
When developing web applications with SvelteKit, understanding how to manage delayed execution can be crucial for enhancing user experience and optimizing server-side processes.
Managing Delays in SvelteKit Actions In a SvelteKit application, server-side logic often resides in endpoints or actions, where you might need to introduce delays for various reasons, such as rate-limiting or simulating long-running processes during development.
Implementing Delays with Promises
and setTimeout
One common approach to introducing a delay on the server side is by using setTimeout
within a Promise
. This method is particularly useful in async
functions where you wish to pause execution for a set period.
// In a SvelteKit endpoint or action
export const actions = {
create: async ({ request }) => {
// Introduce a 5-second delay
await new Promise(resolve => setTimeout(resolve, 5000));
//..rest of your code
}
};
In this example, the
await new Promise(resolve => setTimeout(resolve, 5000));
line effectively pauses the server-side logic for 5 seconds before continuing. This pattern can be especially useful for debugging, testing how your app handles latency, or managing API call rates.
Enhancing UX with JavaScript Delays On the client side, managing delays is often about enhancing user experience. Whether it's showing a spinner while data loads, delaying a notification's disappearance, or throttling rapid user inputs, JavaScript's setTimeout is a valuable tool.
In Svelte and SvelteKit, client-side scripts can utilize setTimeout
directly to manage UI-related delays. Wrapping setTimeout
in a Promise
is a good practice when working within asynchronous functions, allowing you to await the delay.
<script>
import { onMount } from 'svelte';
onMount(async () => {
//rest of your code
// Wait for 3 seconds before executing the next line
await new Promise(resolve => setTimeout(resolve, 3000));
console.log('3 seconds have passed');
});
</script>
This snippet demonstrates how to introduce a delay within the
onMount
lifecycle function of a Svelte component, delaying subsequent operations by 3 seconds.
While introducing delays can be beneficial in many scenarios, it's important to use them judanimately to avoid degrading the user experience. Delays on the client side should be user-centric, enhancing feedback or managing UI states effectively. On the server side, delays can be used for rate limiting or simulating network conditions, but they should be used carefully to avoid unnecessary latency.