Frappe ORM: Working with Documents and Queries
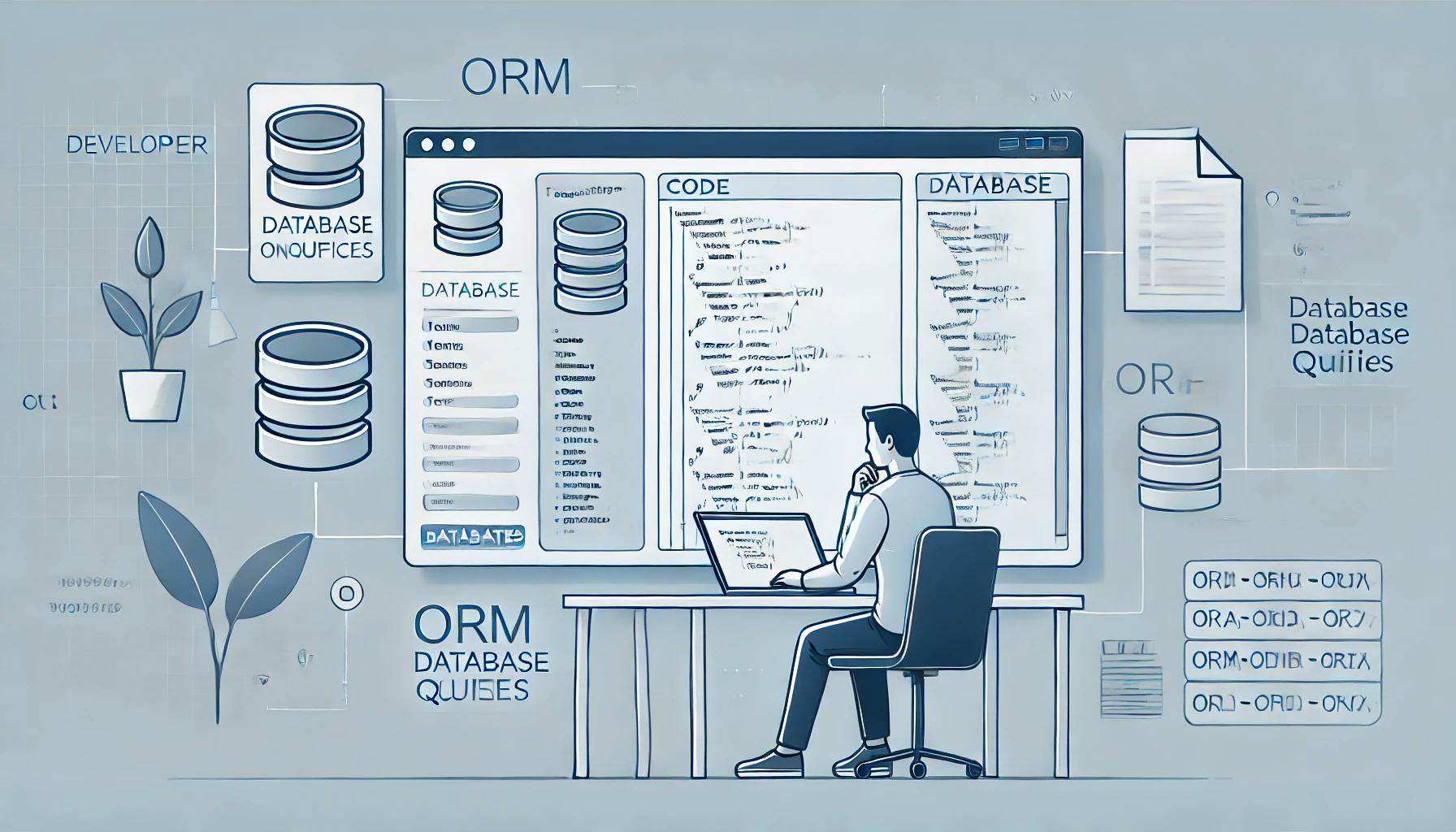
bench --site batsite console --autoreload
Running the above command initially outputs all the installed apps on the site:
Apps in this namespace:
frappe, batwara, rentals
d = frappe.db.get_all('Vehicle')
print(d)
The frappe.db.get_all('Vehicle')
command returns all entry names in the Vehicle
DocType.
for vehicle in vehicles:
print(vehicle)
In [26]: d = frappe.db.get_all('Vehicle', pluck='name')
In [27]: for v in d:
...: vehicle = frappe.get_doc("Vehicle", v)
...: print(vehicle)
...: print("\n")
...: print(f"{vehicle.make} {vehicle.model}")
This example retrieves all vehicles and prints each one along with its make
and model
fields.
In [5]: frappe.db.get_value("Driver", "DR-0001", "first_name")
Out[5]: 'Ferdous'
In this case, we query the Driver
DocType. Here, "DR-0001"
is the document ID, and "first_name"
is the field name being queried.
In [6]: frappe.get_doc("Driver", "DR-0001")
Out[6]: <Driver: DR-0001>
In [7]: d = frappe.get_doc("Driver", "DR-0001")
In [8]: d.first_name
Out[8]: 'Ferdous'
In [9]: d.license_number
Out[9]: 'ERT5739323'
This example shows how to retrieve a specific document by its ID (DR-0001
) from the Driver
DocType. The document is stored in the variable d
, which is then used to access fields like first_name
and license_number
.
In [2]: d = frappe.new_doc("Driver")
In [3]: d.first_name = "Saad"
In [4]: d.license_number = "TRE900"
In [5]: d.save()
Out[5]: <Driver: DR-0003>
In [6]: frappe.db.commit()
In this example, we create a new document inside the Driver
DocType, set the values for first_name
and license_number
, save the document, and finally commit the changes to the database.