Getting Started with Python Classes Methods, Attributes, and Usage
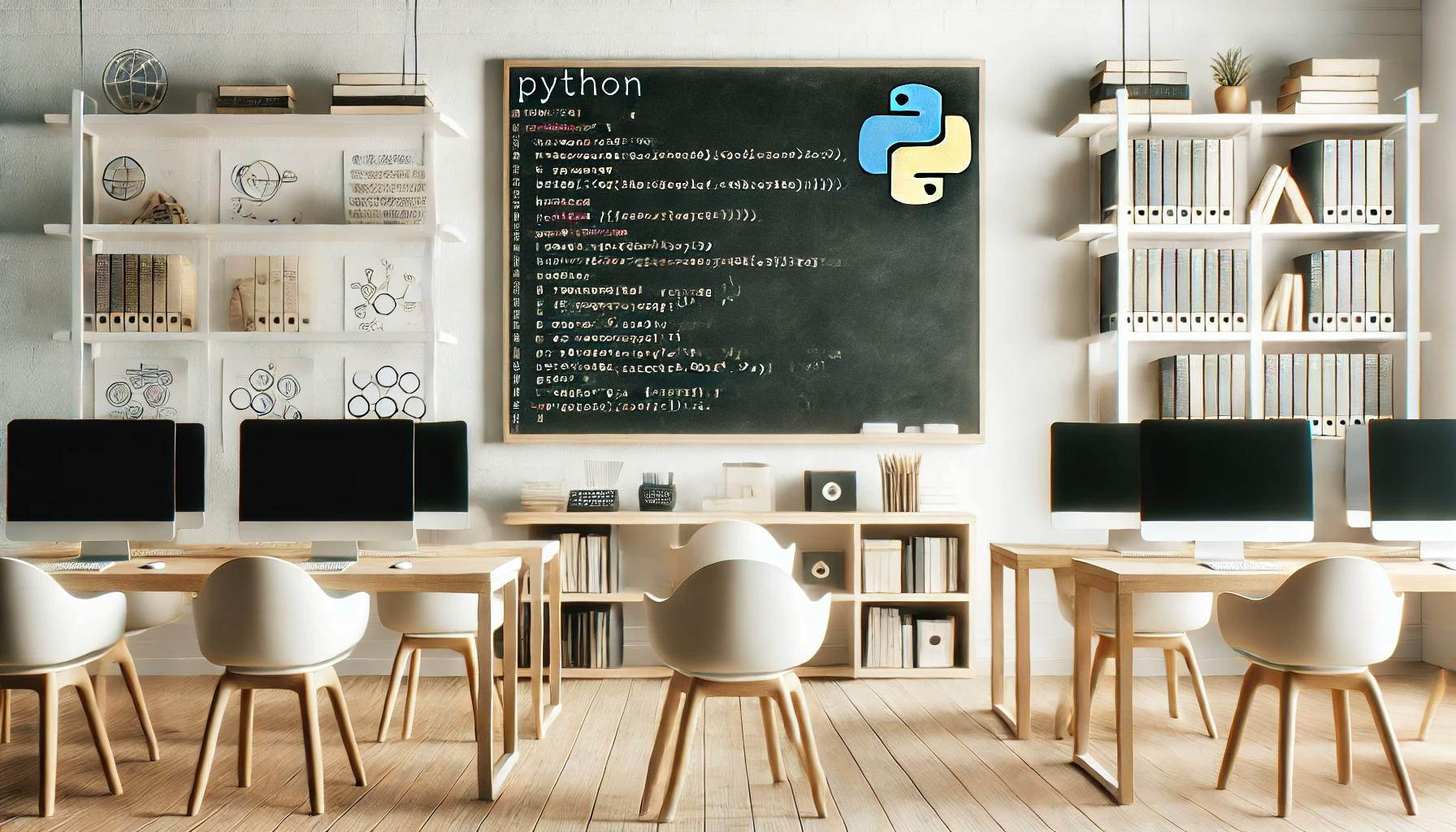
In Python, a class
is a blueprint for creating objects.
This guide demonstrates a basic example of a class
in Python where the properties of a chair, such as legs
and handle
, are defined as attributes, and its functionality is represented by a method called feature
.
class Chair:
legs = 4
handle = 2
def feature(self):
print("You can sit")
In this example:
legs
and handle
are attributes of the Chair
class. lges
is also class variable, becasue this will be available to all of the instance of Chair
class. feature
is a method that describes what the chair can do. Chair
ClassNow, let's create an instance of the Chair
class and use its method.
class Chair:
legs = 4
handle = 2
def feature(self):
print(f"You can sit, and it has {self.legs} legs")
chair_instance = Chair()
chair_instance.feature()
Here:
Chair
class and stored it in chair_instance
. feature
method, which uses f-string
(formatted string literal) to include the number of legs in the output. The __init__
method, also known as the constructor, allows you to set the initial state of an object when it is created.
class Chair:
def __init__(self, name):
self.name = name # The __init__ method initializes the object's state
print(name)
legs = 4
handle = 2
def feature(self):
print(f"You can sit, and it has {self.legs} legs")
chair_instance = Chair('Wooden chair')
chair_instance.feature()
Here:
__init__
method takes self
(reference to the current object) and name
as parameters. name
is an attribute of the Chair
class. For every instance the name will be different and it is instance specific __init__
is called automatically, setting the initial state of the object. Attributes like legs
and handle
are called class attributes because they belong to the class itself, not to any specific instance. This means that all objects created from the Chair
class will share these attributes.
class Chair:
legs = 4
handle = 2
def feature(self):
print("You can sit")
@classmethod
A class method is a method that is bound to the class and not the instance of the class. You can use the @classmethod
decorator to create a class method. Here’s an example:
class Chair:
def __init__(self, name):
self.name = name
print(f"New chair added as {name}")
Chair.add_chair() # Calling the class method
legs = 4
handle = 2
number_of_chair = 0
def feature(self):
print(f"You can sit, and it has {self.legs} legs")
@classmethod
def add_chair(cls):
cls.number_of_chair += 1
print(f"Total number of chairs: {cls.number_of_chair}")
chair_instance1 = Chair('Wooden chair')
chair_instance2 = Chair('Steel chair')
Here:
add_chair
is a class method because it uses the @classmethod
decorator. cls
as an argument, which refers to the class itself (Chair
). Class methods typically perform operations that affect the class as a whole, such as modifying class-level attributes. Output:
New chair added as Wooden chair
Total number of chairs: 1
New chair added as Steel chair
Total number of chairs: 2
def
keyword. Functions can be called independently and are not bound to any object or class.def greet():
print("Hello!")
greet()
self
parameter to refer to the instance calling the method.class Person:
def greet(self): # 'self' refers to the instance calling this method
print("Hello!")
person_instance = Person()
person_instance.greet() # Calling a method