How to Calculate and Normalize SVG Path Length in JavaScript
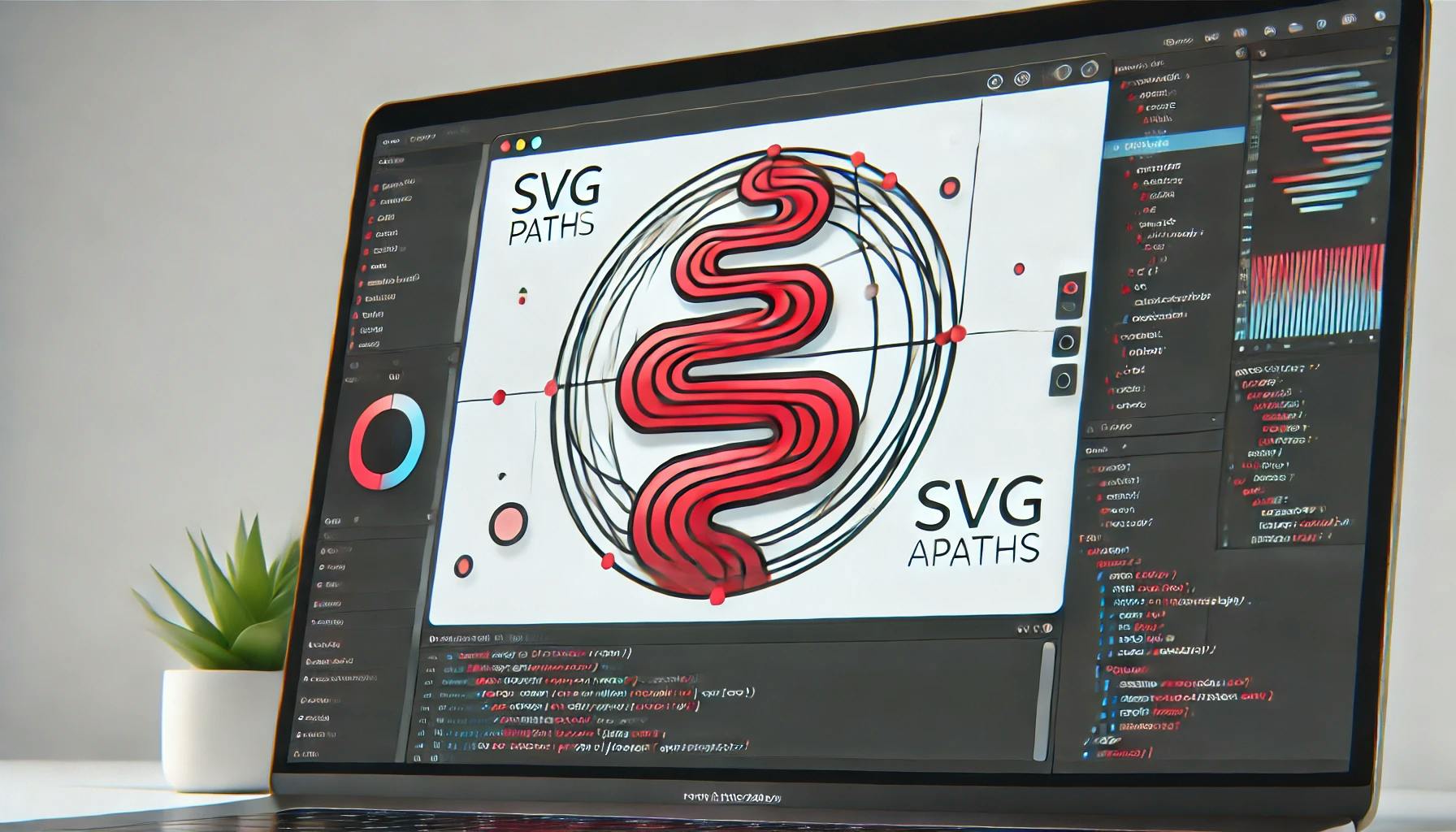
To begin, right-click on the SVG path element and select Inspect in your browser’s developer tools (usually, pressing F12
or Ctrl+Shift+I
opens the developer tools). This will open the Elements tab, where you can see the underlying HTML code for the SVG.
Next, switch to the Console tab in the developer tools. Here, you can execute JavaScript code that interacts with the page. If you type $0
and press Enter, you'll see the output of the currently selected element (in this case, the SVG path element).
Now, to get the total length of the SVG path, you can run the following JavaScript command:
$0.getTotalLength();
This will return the total length of the path in user units (typically pixels).
By default, every SVG
has a different path length
, and managing this can become difficult.
Thankfully, there's a way to normalize
the length of the SVG path
by defining the path length
as percentage
or a fixed unit
.
Here’s an example with a basic SVG
path:
<svg viewBox="0 0 1087 408">
<path
class="fill-none stroke-red-500 stroke-[1px]"
d="M0.5 139C90 68.5 287 -52.7 359 26.5C449 125.5 297.5 268.5 461.5 251.5C625.5 234.5 555 102 766.5 120.5C935.7 135.3 837 318 766.5 407.5L1086.5 97"
/>
</svg>
To get the length of this path, you would again use the getTotalLength()
method.
Now, to normalize the path length to a specific value (e.g., 1), you can add the pathLength
attribute directly to the <path>
element:
<svg viewBox="0 0 1087 408">
<path
class="fill-none stroke-red-500 stroke-[1px]"
pathLength="1"
d="M0.5 139C90 68.5 287 -52.7 359 26.5C449 125.5 297.5 268.5 461.5 251.5C625.5 234.5 555 102 766.5 120.5C935.7 135.3 837 318 766.5 407.5L1086.5 97"
/>
</svg>
By setting pathLength="1"
, you’re telling the browser to treat the path as if it has a total length of 1 unit. This normalization is particularly useful when animating the path, such as when you want to create an animation that "draws" the path over time (using CSS or JavaScript). It makes the animation easier to manage since the path length is consistent.
Note that even though the path length is normalized for animations, the value returned by getTotalLength()
will remain the same as the original length.