How to Implement Apache ECharts in Reactjs app
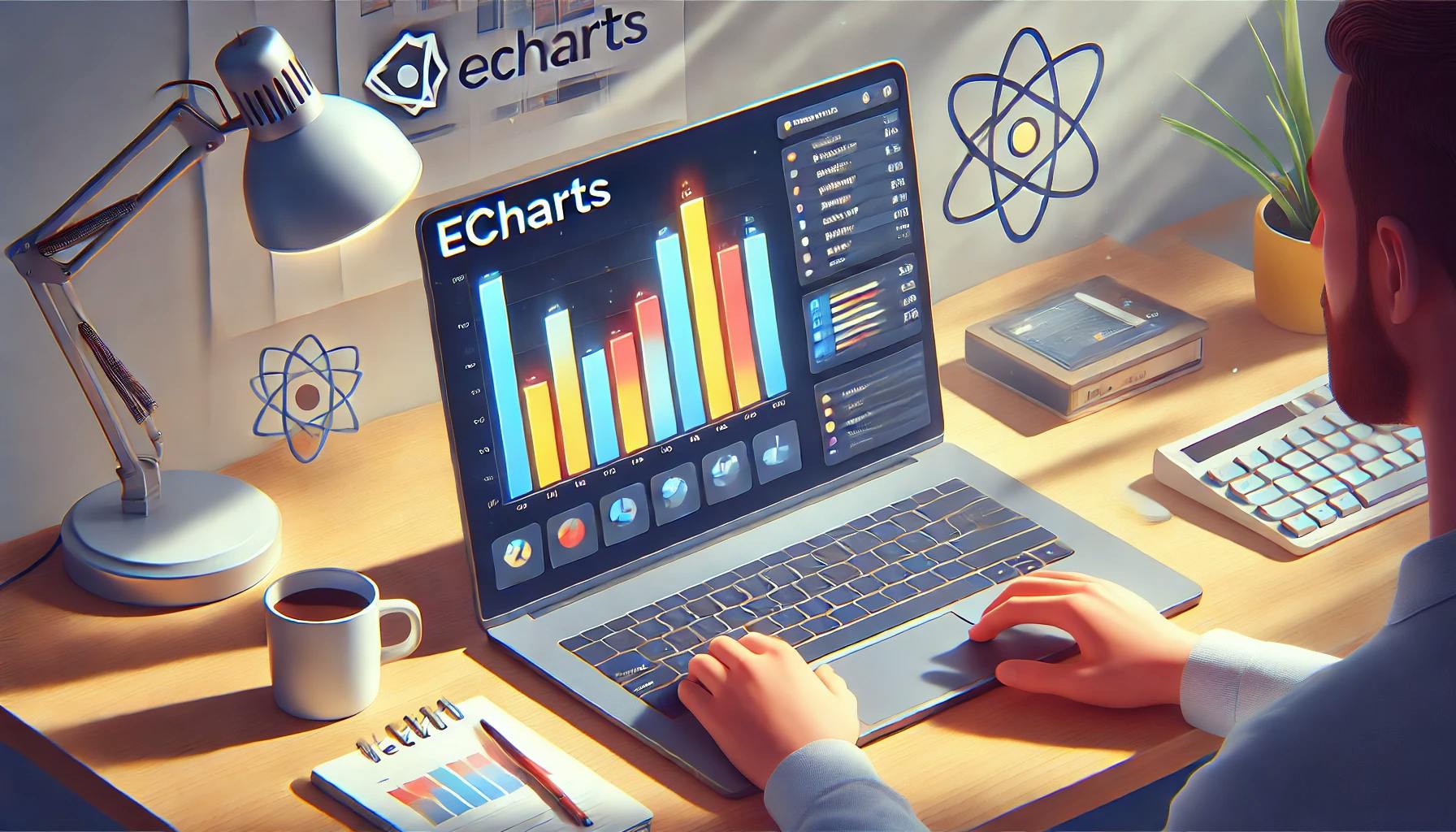
Apache ECharts
is a powerful charting library that can be easily integrated into a React
application.
In this guide, we'll walk through the 3 fundamental steps required to render an ECharts chart in your React app.
div
element. In react
we can use useRef
to store a reference of the div
element options
object is passed to the chart instance using chartInstance.setOption(options)
Let’s comply with all the required steps and implement them in the React
app
If you want to install
react
app efficiently withtailwindcss
&daisyui
, you can follow my blogpost Install React Efficiently (react + vite + tailwindcss + daisyui)
ECharts
with a DOM element, which can be done by referencing a div
element.
In React, we use the useRef
hook to store a reference to this div
.import { useEffect, useRef } from "react";
import * as echarts from "echarts";
const ChartOne = () => {
const chartRef = useRef(null);
useEffect(() => {
console.log(chartRef);
}, []);
return (
<div ref={chartRef} style={{ width: "600px", height: "400px" }}></div>
);
};
export default ChartOne;
In the code above, we created a reference to the
div
element usinguseRef
and logged it to the console to verify.
options
. ECharts
uses an options
object to configure various aspects of the chart, such as the title, axes, series data, tooltips, and legends. We'll pass this options
object to the chart instance using chartInstance.setOption(options)
.import { useEffect, useRef } from "react";
import * as echarts from "echarts";
const ChartOne = () => {
// console.log(echarts);
const chartRef = useRef(null);
useEffect(() => {
console.log(chartRef);
// initialize the chart instance
const chartInstance = echarts.init(chartRef.current);
// Define the chart options
const options = {
title: {
text: "Echarts Example",
},
};
// Adding the options to the chart
chartInstance.setOption(options);
}, [chartRef]);
return (
<div ref={chartRef} style={{ width: "600px", height: "400px" }}></div>
);
};
export default ChartOne;
title
rendered on the screen. options
object. The data is typically included within the series
property of the options, and its format depends on the chart type (e.g., line, bar, pie).// Define the chart options
const options = {
title: {
text: "Echarts Example",
},
xAxis: {
data: ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"],
},
yAxis: {},
series: [
{
name: "Sales",
type: "bar",
data: [5, 20, 36, 10, 10, 20],
},
],
};
With this setup, you should now see the chart rendered with the provided data. However, you might encounter warnings related to React's strict mode.
chart instance
is properly managed, we'll add a cleanup function that disposes
of the chart
instance when it's no longer needed.import { useEffect, useRef } from "react";
import * as echarts from "echarts";
const ChartOne = () => {
const chartRef = useRef(null);
const chartInstanceRef = useRef(null); // Ref to hold the chart instance
useEffect(() => {
console.log(chartRef);
// Initialize a new chart instance
chartInstanceRef.current = echarts.init(chartRef.current);
// Define the chart options
const options = {
title: {
text: "Echarts Example",
},
xAxis: {
data: ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"],
},
yAxis: {},
series: [
{
name: "Sales",
type: "bar",
data: [5, 20, 36, 10, 10, 20],
},
],
};
// Apply the options to the chart
chartInstanceRef.current.setOption(options);
// Cleanup function to dispose of the chart instance
return () => {
chartInstanceRef.current.dispose();
};
}, [chartRef]);
return (
<div ref={chartRef} style={{ width: "600px", height: "400px" }}></div>
);
};
export default ChartOne;
Now, your chart should render without any warnings or errors, providing a clean and smooth experience.