NGINX Beginner’s Guide Web Server & Load Balancer Explained
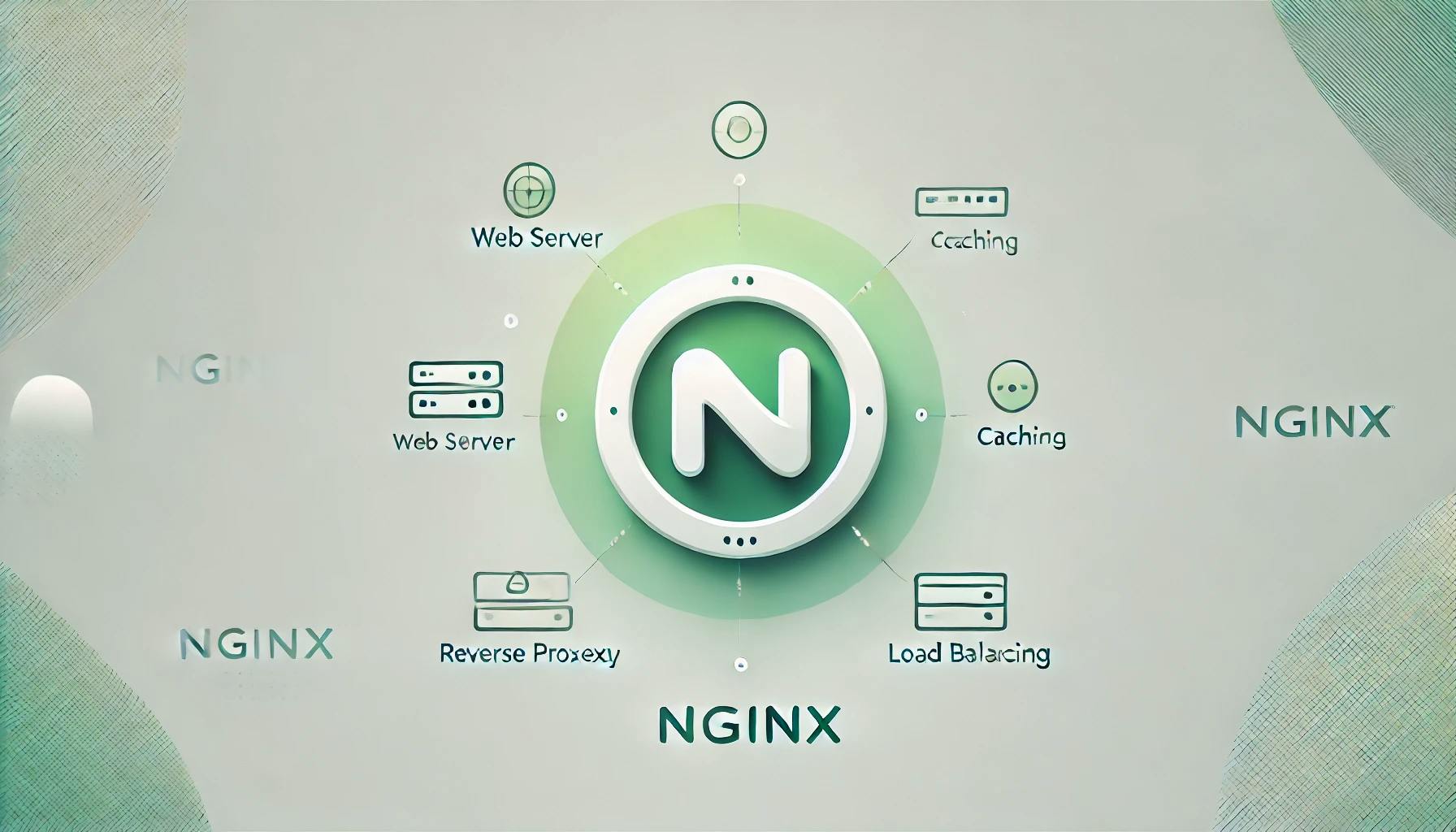
NGINX (pronounced "engine-X") is a powerful and efficient web server and reverse proxy that handles HTTP requests. It serves as the first layer in a web application, sitting between clients (browsers) and backend servers, routing requests efficiently.
NGINX is commonly used for:
NGINX is optimized for handling multiple concurrent connections, making it a preferred choice for high-traffic websites and cloud-based applications.
NGINX efficiently processes and routes HTTP requests, ensuring optimal web performance.
NGINX distributes incoming traffic across multiple servers to improve reliability and reduce downtime.
There are two primary load-balancing methods:
In environments with multiple backend servers, managing direct public access can be complex and risky. Instead, one NGINX server can act as the primary gateway, handling all requests and distributing them securely to backend servers.
Benefits of using NGINX as a proxy server:
✅ Enhanced Security – Hides backend servers from public access.
✅ Simplified Management – Centralized request routing.
✅ Load Balancing – Optimizes server resources.
NGINX stores cached responses, reducing server load and improving performance.
NGINX compresses files before transmission and supports chunked video streaming to reduce bandwidth costs.
NGINX acts as an SSL/TLS terminator by handling encrypted HTTPS requests:
The main configuration file is nginx.conf
, typically located at /etc/nginx/nginx.conf
.
server {
listen 80;
server_name example.com www.example.com;
location / {
root /var/www/example.com;
index index.html index.htm;
}
}
server {
listen 80;
server_name example.com www.example.com;
location / {
proxy_pass http://backend-server-ip:port;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
server {
listen 443 ssl;
server_name example.com www.example.com;
ssl_certificate /etc/nginx/ssl/cert.pem;
ssl_certificate_key /etc/nginx/ssl/key.pem;
ssl_protocols TLSv1.2 TLSv1.3;
ssl_ciphers 'ECDHE-RSA-AES128-GCM-SHA256:ECDHE-RSA-AES256-GCM-SHA384';
location / {
root /var/www/example.com;
index index.html index.htm;
}
}
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
listen 80;
server_name lb.example.com;
location / {
proxy_pass http://backend;
}
}
upstream backend {
least_conn;
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
http {
proxy_cache_path /data/nginx/cache levels=1:2 keys_zone=my_cache:10m max_size=1g inactive=60m;
server {
listen 80;
server_name example.com www.example.com;
location / {
proxy_pass http://backend-server-ip;
proxy_cache my_cache;
proxy_cache_valid 200 302 10m;
proxy_cache_valid 404 1m;
}
}
}