React Page Navigation with React Router
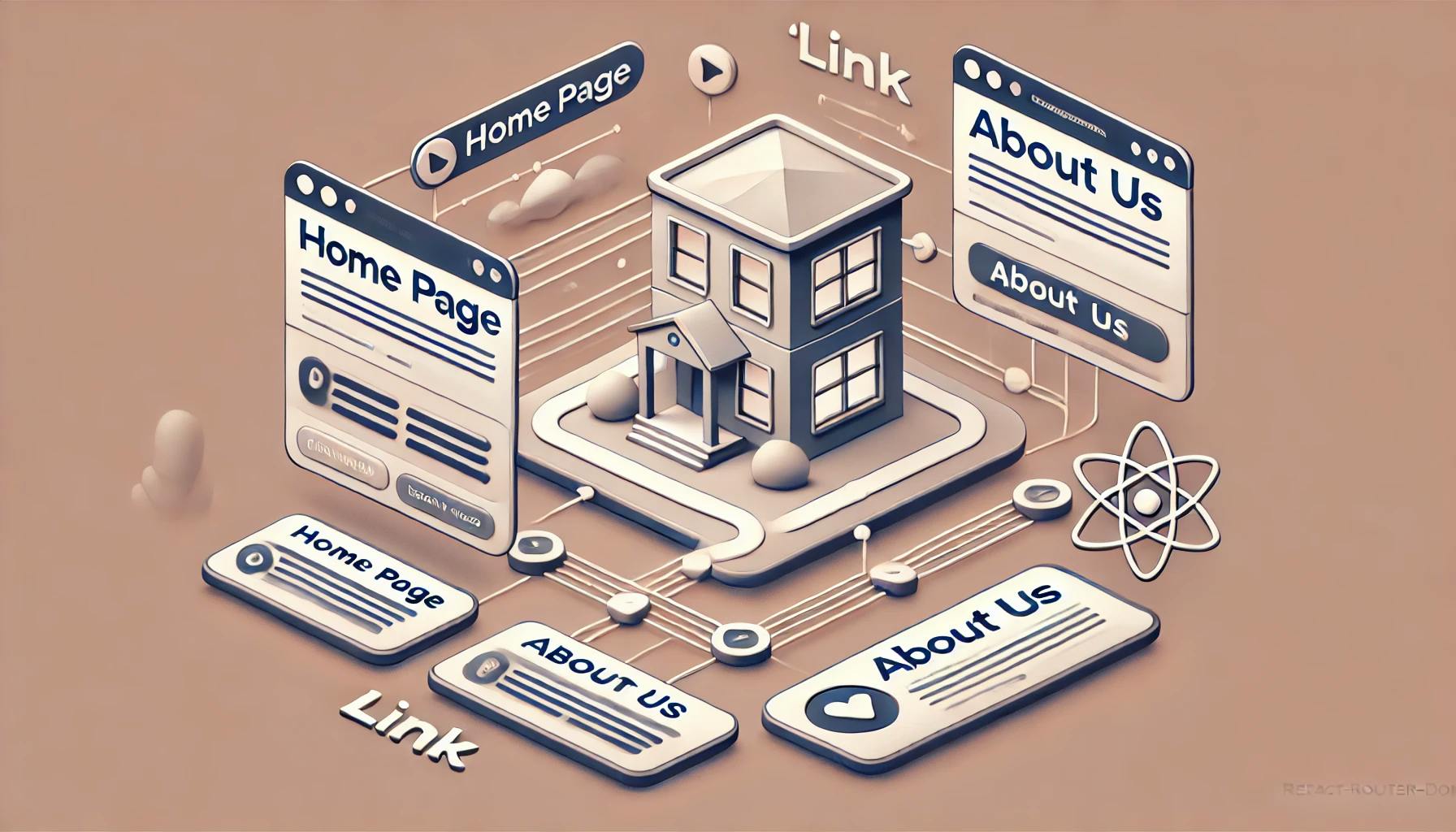
The react-router
package is the heart of React Router
and provides all the core functionality for both react-router-dom
and react-router-native
.
While react-router-dom
is designed for web applications and react-router-native
is for React Native mobile apps.
You can follow my blogpost to Create a react project quickly with tailwindcss and daisyui.
react-router-dom
npm i react-router-dom
Let’s create a folder named pages
inside the src
folder.
Now, create two files AboutUsPage.jsx
and HomePage.jsx
insiside the pages
folder.
Here is the code for HomePage.jsx
and AboutUsPage.jsx
:
function HomePage() {
return (
<>
<title>React Learning: Home Page</title>
<div className="py-5">
<div className="flex justify-center flex-col gap-2">
<h1>Hey, let's navigate between pages</h1>
<p>
Go to{" "}
<a className="btn btn-primary" href="/aboutUs">
About Us ➙
</a>{" "}
page
</p>
</div>
</div>
</>
);
}
export default HomePage;
function HomePage() {
return (
<>
<title>React Learning: About Us Page</title>
<div className="py-5">
<div className="flex justify-center flex-col gap-2">
<h1>Hey, let's navigate between pages</h1>
<p>
Go to{" "}
<a className="btn btn-primary" href="/">
Home ➙
</a>{" "}
page
</p>
</div>
</div>
</>
);
}
export default HomePage;
Now, open the app.jsx
file and add both of the page components to the App
function.
import "./App.css";
import HomePage from "./pages/HomePage";
import AboutUsPage from "./pages/AboutUsPage";
function App() {
return (
<>
<title>React Learning</title>
<div className="flex justify-center flex-col gap-2">
<h1 className="text-3xl">Hello React World!</h1>
</div>
<HomePage />
<AboutUsPage />
</>
);
}
export default App;
Import BrowserRouter
, Route
, and Routes
from react-router-dom
in the App.jsx
file:
import { BrowserRouter, Route, Routes } from "react-router-dom";
Now, use these components inside the App
function:
<BrowserRouter>
<Routes>
<Route index element={<HomePage />} />
<Route path="/home" element={<HomePage />} />
<Route path="/aboutUs" element={<AboutUsPage />} />
</Routes>
</BrowserRouter>
In this setup, we set <HomePage />
as the default route.
If you test the code in the browser, you may notice that the entire page reloads each time we navigate to a different page. This happens because we used the default HTML anchor link
<a href=""></a>
.
To prevent this behavior, let's improve the HomePage
and AboutUsPage
code.
Update the anchor
tag
<a className="btn btn-primary" href="/aboutUs">
About Us ➙
</a>
With Link
component, imported from react-router-dom
<Link className="btn btn-primary" to="/aboutUs">
About Us ➙
</Link>
Here is the complete code for HomePage.jsx
import { Link } from "react-router-dom";
function HomePage() {
return (
<>
<title>React Learning: Home Page</title>
<div className="py-5">
<div className="flex justify-center flex-col gap-2">
<h1>Hey, lets navigate between pages</h1>
<p>
Go to
<Link className="btn btn-primary" to="/aboutUs">
About Us ➙
</Link>
page
</p>
</div>
</div>
</>
);
}
export default HomePage;
Repeat the same step for the AboutUsPage
as well.
Now, if you check in the browser, you will notice that the page no longer reloads when navigating between pages.