Simplifying Store vs State Management in Web Apps with Examples
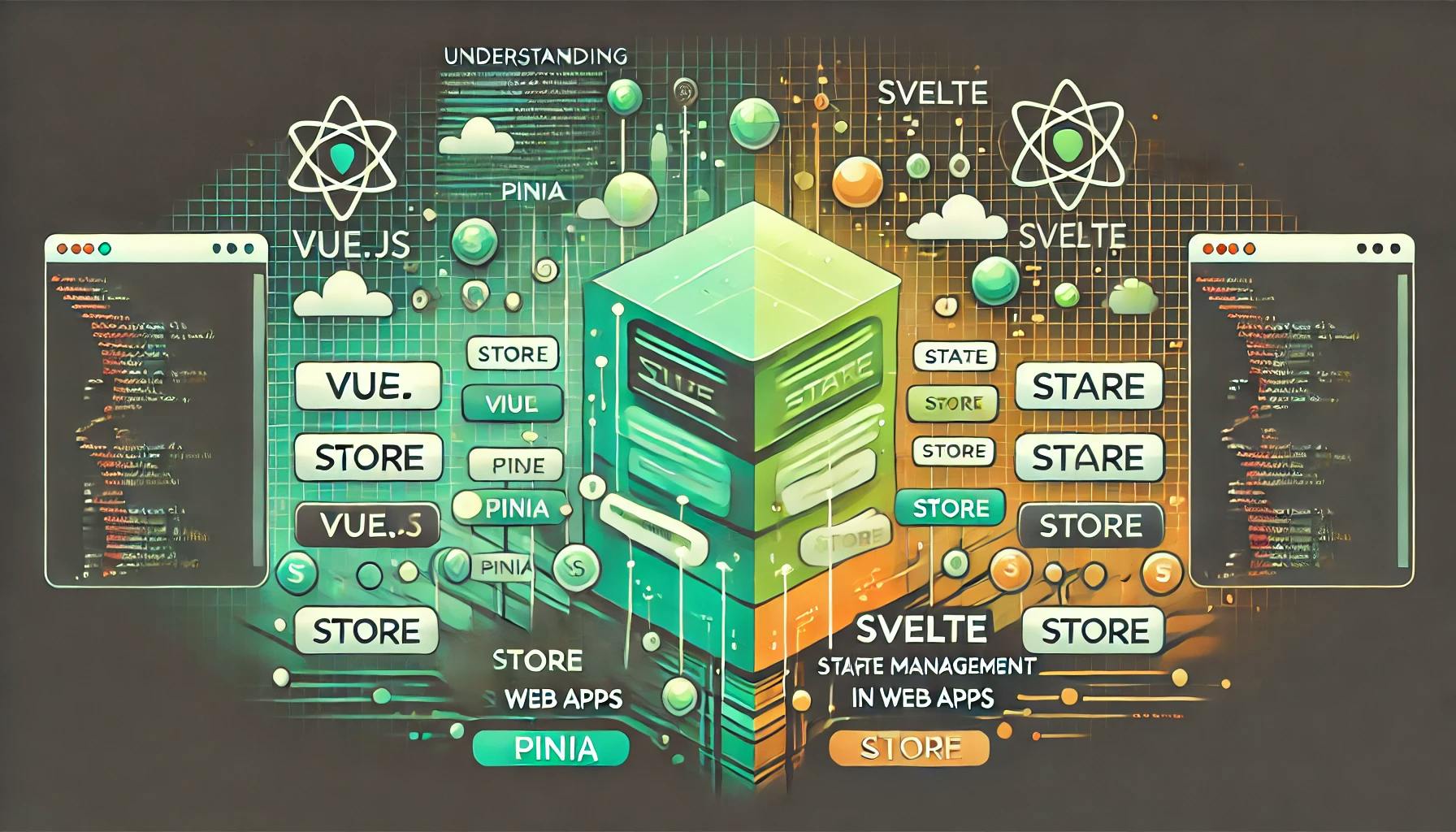
Understanding store
and state management
can significantly enhance your ability to design and manage web applications effectively.
These terms, while related, address different aspects of handling data within an application.
Here’s a straightforward explanation with examples from popular frameworks like Vue
(using Pinia
) and Svelte
.
State management
refers to overseeing the data
("state") within your application throughout its lifecycle. This encompasses user data, settings, UI state, and any changes to this data. Proper state management
ensures that your app responds dynamically to interactions and maintains consistent behavior.
Key aspects of state management include:
A store
is a specific implementation within state management
frameworks that serves as a centralized container for the application's state. It ensures that the state is managed in a unified and predictable manner, improving both performance and developer experience.
Characteristics of a store:
Pinia
is used for state management in Vue.js
applications. It provides a centralized store
that manages the state of the entire application or significant parts of it.import { defineStore } from "pinia";
export const useUserStore = defineStore("user", {
state: () => ({
name: "Alice",
age: 30,
}),
actions: {
birthday() {
this.age++;
},
},
});
In this example,
useUserStore
is aPinia
store
with user details and an action to increment the user's age.
store
is a simple but powerful state management
solution native to Svelte
. It allows components to subscribe
to state changes
efficiently.import { writable } from "svelte/store";
const count = writable(0);
function increment() {
count.update((n) => n + 1);
}
Here,
count
is areactive store
that can be updated and subscribed to across components.
reactivity
using reactive variables
declared with the $:
syntax. This approach is great for automatic updates within components.<script>let count = 0; $: doubled = count * 2;</script>
doubled
automatically updates whenever count changes, showcasing Svelte's reactive capabilities.
Consider using a store
when dealing with complex data interactions, requiring debugging tools, or managing shared state across multiple components.
For simpler data needs, direct state management
(like Svelte's reactive variables or Vue's local component state) might suffice.
Differentiating between store
and state management
helps structure your web applications more effectively. Understanding when and how to use tools like Pinia or Svelte Store can make your development process smoother and your applications more robust and responsive.