Svelte 5 Reactive Statements vs Svelte 4 Reactive Statements
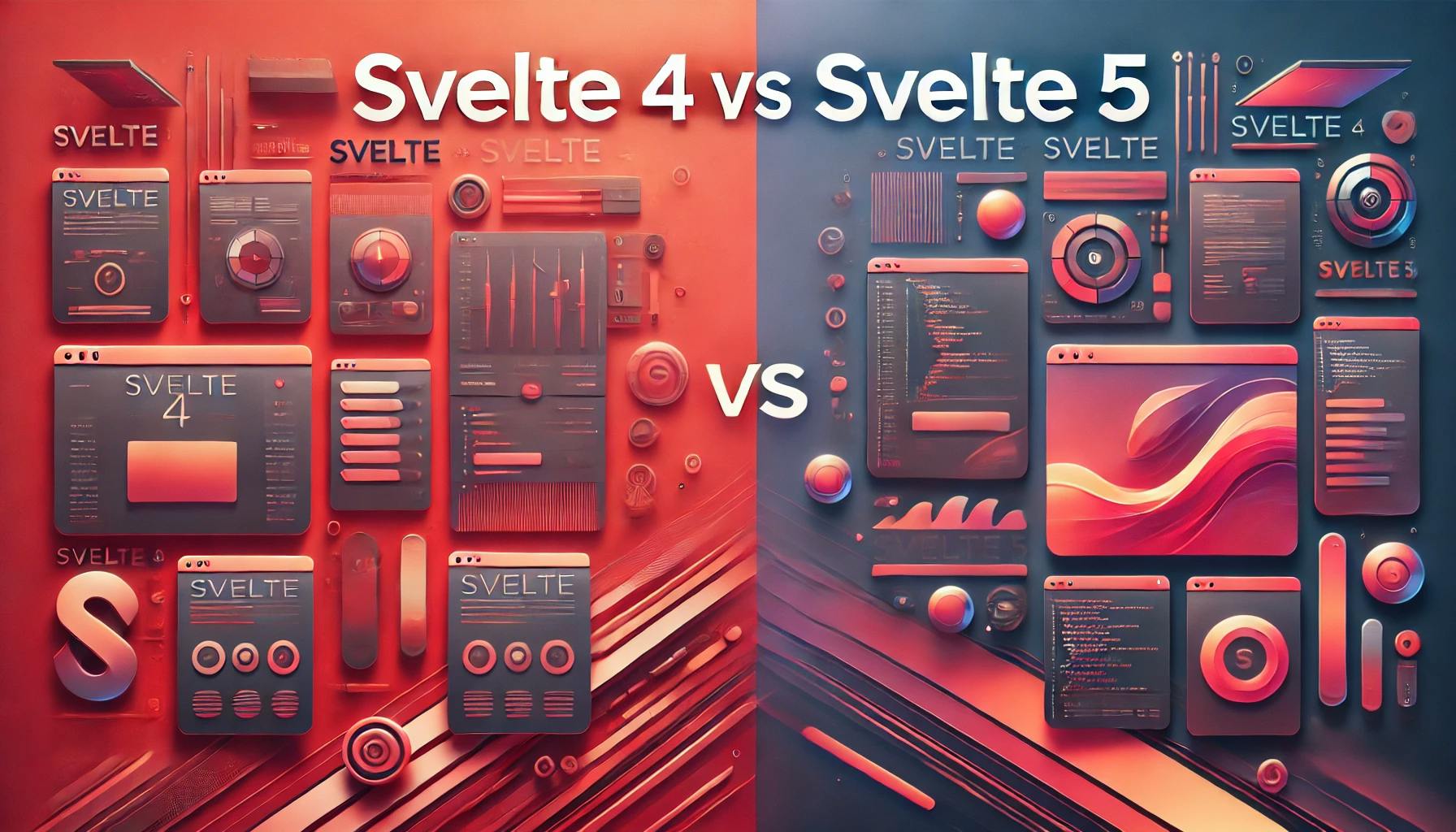
Svelte, a popular front-end framework, has always been celebrated for its unique approach to reactivity. With the introduction of Svelte 5, the framework has refined its reactive statement model, taking it a step further to offer developers more clarity, power, and control. Here, we delve into the differences between Svelte 4 and Svelte 5’s reactive statements, highlighting how they cater to modern development needs.
In Svelte 4, reactivity was driven by the $:
syntax. This declarative approach allowed developers to define reactive statements and variables that automatically updated whenever the dependencies changed.
<script>
let count = 0;
$: double = count * 2;
function increment() {
count += 1;
}
</script>
<p>{count}</p>
<p>{double}</p>
<button on:click="{increment}">Increment</button>
Here, the $:
syntax ensures that the double
variable is recalculated every time count
changes. This model is straightforward and integrates seamlessly with Svelte’s compiler-driven updates, but it does have limitations, particularly in complex scenarios where managing dependencies explicitly can become cumbersome.
Feature | Svelte 4 | Svelte 5 |
---|---|---|
Syntax and Explicitness | $: syntax for implicit reactivity | Runes like $state and $derived for explicit reactivity |
Granularity | Compiler determines dependencies automatically | Developers define reactive dependencies explicitly |
Debugging and Maintenance | Challenging in complex dependency scenarios | Easier with explicit state and derived declarations |
Compatibility and Learning Curve | Beginner-friendly and quick to adopt | Slight learning curve but better for complex apps |
Svelte 5 introduces a new concept known as "runes," which redefine how reactivity is handled. Runes, such as $state
and $derived
, provide a more explicit and controlled mechanism for declaring reactive variables and derived states. This shift offers developers a better understanding of data flow and more granular control over reactivity.
<script>
let count = $state(0);
const double = $derived(() => count * 2);
function increment() {
count += 1;
}
</script>
<p>{$count}</p>
<p>{$double}</p>
<button on:click="{increment}">Increment</button>
Here, count
is explicitly defined as a reactive state using $state
, and double
is a derived value that automatically updates whenever count
changes. By using runes, Svelte 5 separates the declaration of state from its derived calculations, making the logic more explicit and easier to debug.
<script>
let numbers = $state([1, 2, 3]);
const sum = $derived(() => numbers.reduce((a, b) => a + b, 0));
function addNumber() {
numbers = [...numbers, numbers.length + 1];
}
</script>
<p>Numbers: {$numbers.join(', ')}</p>
<p>Sum: {$sum}</p>
<button on:click="{addNumber}">Add Number</button>
This example demonstrates how arrays can be managed reactively with $state
and $derived
.
<script>
let isLoggedIn = $state(false);
const statusMessage = $derived(() =>
isLoggedIn ? "Welcome back!" : "Please log in."
);
function toggleLogin() {
isLoggedIn = !isLoggedIn;
}
</script>
<p>{$statusMessage}</p>
<button on:click="{toggleLogin}">{$isLoggedIn ? 'Log Out' : 'Log In'}</button>
This example shows conditional reactivity, where the statusMessage
updates based on the isLoggedIn
state.
<script>
let user = $state({ name: "John", age: 30 });
const greeting = $derived(() => `Hello, ${user.name}!`);
function updateName(newName) {
user = { ...user, name: newName };
}
</script>
<p>{$greeting}</p>
<input type="text" placeholder="Enter name" on:input={(e) =>
updateName(e.target.value)} />
This example highlights how objects can be used reactively
, updating the greeting whenever the user.name
changes.