Sveltekit Change Page Title Dynamically
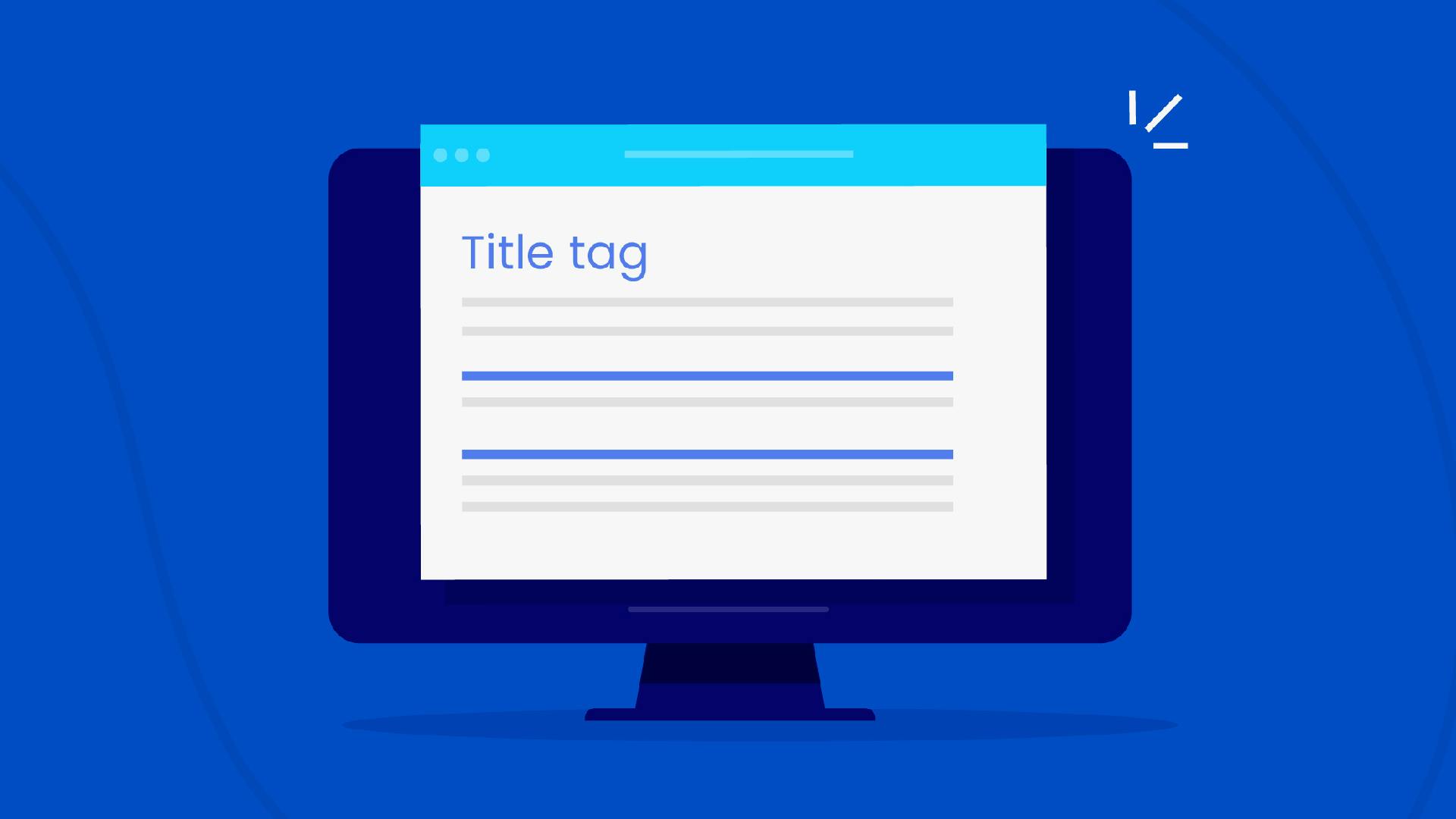
The <svelte:head>
is a special element you can use to insert elements inside the <head>
element of a webpage.
You can add this to any page and set the page name like so:
<svelte:head>
<title>Tutorial</title>
</svelte:head>
pathname
)Setting the page name in a static manner can be a repetitive process. We can improve this by using the +layout.svelte
file.
+layout.svelte
& import page
from $app/stores
import { page } from "$app/stores";
Console log these values to understand what the page
store is holding$: console.log("page info is: ");
$: console.log($page);
$: console.log($page.params.b);
$: console.log($page.route.id);
$: console.log($page.url.pathname);
$page.url.pathname
output $page.url.pathname
output consists of a ‘/’
at the beginning, to remove that we can use the JavaScript slice(1)
function, which will slice and remove the first character <svelte:head>
<title>Tutorial | {$page?.url?.pathname?.slice(1)}</title>
</svelte:head>
Interpret the code like this - The expression
$page?.url?.pathname
will first check if$page
is defined. If$page
exists, it then checks if$page.url
is defined. Finally, if both$page
and$page.url
is present, it will access the pathname property of$page.url
If at any point in this chain, something is
undefined
ornull
, the entire expression will safely returnundefined
without throwing an error, thanks to the optional chaining operator
<svelte:head>
<title>
Tutorial {$page?.url?.pathname != '/' ? `|
${$page?.url?.pathname?.slice(1)}` : ''}
</title>
</svelte:head>
Interpret the code like this - if
$page?.url?.pathname
value is NOT same as ‘/’ then print the$page?.url?.pathname
value and slice the first character, if not then print blank string ‘ ’