SvelteKit Explained +server.js vs +page.server.js vs +page.js
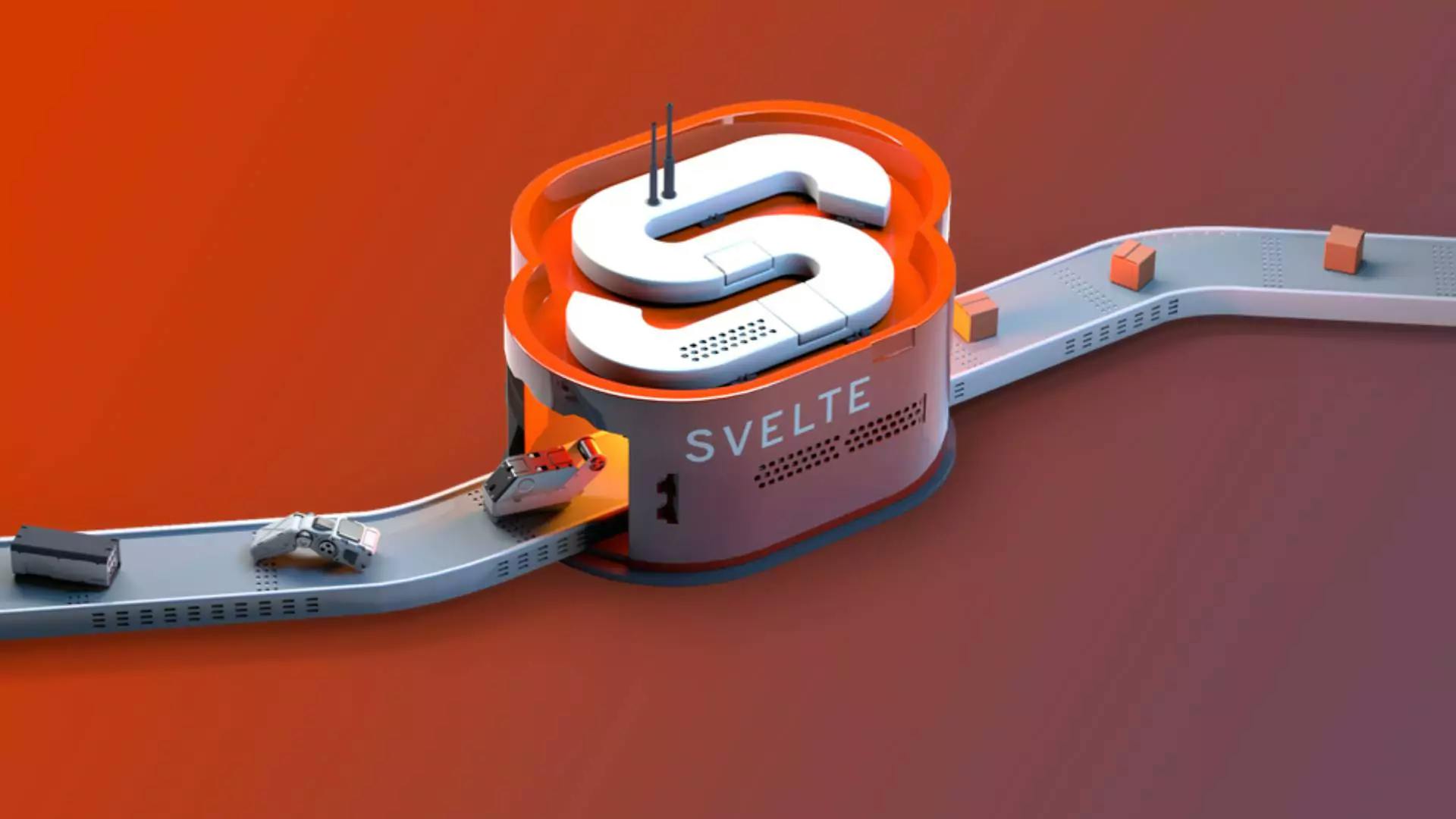
+server.js
, +page.server.js
, and +page.js
ExplainedSvelteKit provides a powerful system for managing both server-side and client-side data fetching through three key files: +server.js
, +page.server.js
, and +page.js
. Each plays a distinct role in handling data and optimizing performance.
+server.js
- Standalone API Routes+server.js
is used for handling server-side logic that isn’t directly tied to a page. It allows you to create API endpoints within your SvelteKit project.
Runs only on the server.
/api/data
). Returns non-HTML responses (e.g., JSON, XML).
Located at src/routes/api/data/+server.js
, it could look like this:
export function GET() {
return new Response(JSON.stringify({ message: 'Hello from API' }), {
headers: { 'Content-Type': 'application/json' }
});
}
+page.server.js
- Server-Side Data Fetching for PagesHandles server-side logic specific to a page, supporting SSR (Server-Side Rendering) and SEO-friendly content generation.
Runs only on the server.
Passes data to +page.svelte
for HTML rendering.
Located at src/routes/blog/[slug]/+page.server.js
:
export async function load({ params }) {
const post = await fetchPostFromDatabase(params.slug);
return { post };
}
+page.js
- Universal Data Fetching (Client & Server)+page.js
provides a universal loader for fetching data on both the server (initial load) and client (subsequent navigation).
Runs on both the server and client.
Passes data to +page.svelte
for HTML rendering.
Located at src/routes/blog/[slug]/+page.js
:
export async function load({ fetch, params }) {
const response = await fetch(`/api/posts/${params.slug}`);
const post = await response.json();
return { post };
}
Note: When a page is first loaded,
+page.js
runs on the server. When navigating between pages via client-side routing, it runs on the client.
+server.js
, +page.server.js
, and +page.js
Feature | +server.js | +page.server.js | +page.js |
---|---|---|---|
Purpose | Standalone server-side API or logic | Server-side data fetching for specific pages | Universal data fetching for server/client |
Execution Context | Server-only | Server-only | Server and client |
Data Fetching | Server-side, non-page related | Server-side, tied to specific pages | Client-side and server-side |
Rendering | Returns non-HTML content | Used with +page.svelte for HTML rendering | Used with +page.svelte for HTML rendering |
Use Cases | API endpoints, file uploads, etc. | SSR data fetching, SEO-focused rendering | Data fetching for both SSR and CSR |
+server.js
when creating API endpoints, handling authentication, or processing server-side tasks unrelated to a specific page. +page.server.js
when you need server-only data fetching for a specific page (e.g., fetching protected or sensitive data). +page.js
for data that should be accessible on both server and client, supporting smooth client-side navigation. By structuring your SvelteKit project properly with these files, you can optimize performance, ensure security, and enhance user experience.