Trigger GitHub Actions with the REST API
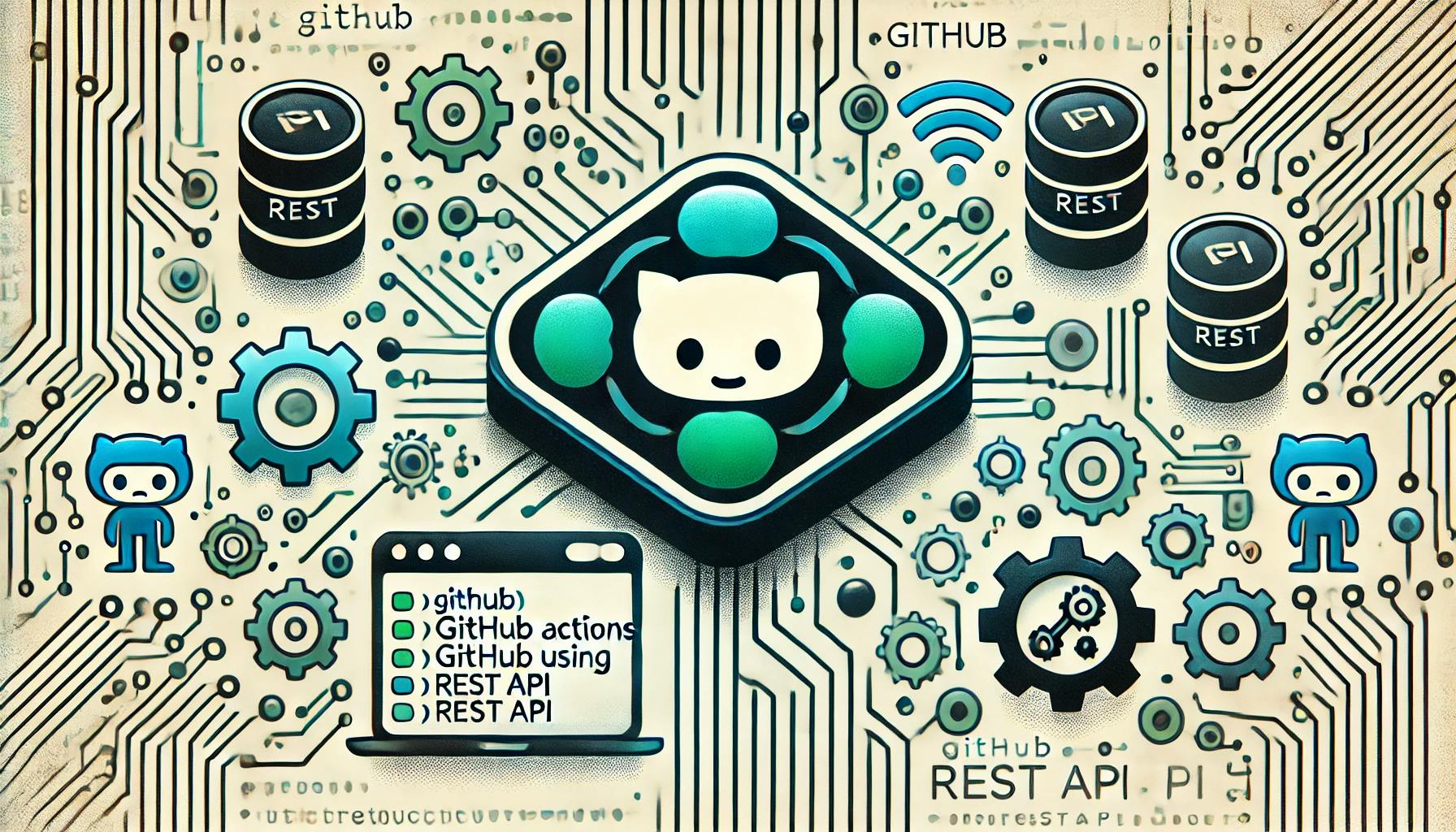
My previous blog post was about how you can write GitHub
Actions
that run every time we push a commit.
In this blog post, we'll explore how to trigger a GitHub
workflows Actions
using the GitHub
REST API
. This method allows you to initiate workflows
programmatically, providing flexibility for integration with third-party applications.
Steps for Triggering GitHub Actions with the REST API
code
rest api
documentation cURL
Command REST API
using Thunder Client
or Postman
code
Here's an example of a simple GitHub Actions workflow configured in the yml
file, that we have written in my previous blogpost
name: Hello World Workflow
on:
push:
branches:
- main
pull_request:
branches:
- main
workflow_dispatch:
jobs:
hello:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: hello world
run: echo "Hello World"
shell: bash
thankyou:
runs-on: ubuntu-latest
steps:
- name: thank you
run: echo "Thank you"
shell: bash
on
event lets us configure when we should run the jobs
workflow_dispatch
parameter inside on
event means we can trigger this workflow from any dispatch
event called using GitHub
rest API
rest api
documentationLet's check the documentation on the GitHub
about the rest api for github workflow
According to the GitHub documentation. we should first use the cURL
structure, to check if everything is working fine, then we can use the rest api
curl -L \
-X POST \
-H "Accept: application/vnd.github+json" \
-H "Authorization: Bearer <YOUR-TOKEN>" \
-H "X-GitHub-Api-Version: 2022-11-28" \
https://api.github.com/repos/OWNER/REPO/actions/workflows/WORKFLOW_ID/dispatches \
-d '{"ref":"topic-branch","inputs":{"name":"Mona the Octocat","home":"San Francisco, CA"}}'
So the requirements are
<YOUR-TOKEN>
: Which we can obtain from Developer Settings
in GitHub
.Settings
Developer Settings
Personal access Tokens
then Fine-grained tokens
and then Generate new token
Actions
repository permissions (write)
WORKFLOW_ID
: File name
of the action *.yml
REPO
- The Repository nameOWNER
- The owner
username of the github
repo ref
- The branch name
of the repo
After obtain all the information the final code should be like this
curl -L ^
-X POST ^
-H "Accept: application/vnd.github+json" ^
-H "Authorization: Bearer ghp_y2Oht7wglnJdBSW" ^
-H "X-GitHub-Api-Version: 2022-11-28" ^
https://api.github.com/repos/sabbirkuasha/sabbirz_sitemap/actions/workflows/sitemap.yml/dispatches ^
-d "{\"ref\":\"main\"}"
Windows user should use
^
("caret" symbol) to be able to run multiline command in terminal
sabbirhossain@Sabbirs-MacBook-Air ~ % curl -L \
-X POST \
-H "Accept: application/vnd.github+json" \
-H "Authorization: Bearer ghp_y2Oht7wglnJdBSW" \
-H "X-GitHub-Api-Version: 2022-11-28" \
https://api.github.com/repos/sabbirkuasha/sabbirz_sitemap/actions/workflows/sitemap.yml/dispatches \
-d '{"ref":"main"}'
macOS user should use
\
("backslash" symbol) to be able to run multiline command in terminal
If you run this code and check on the GitHub
workflows, you should see that you can fire github workflow
using cURL
REST API
using Thunder Client
I am showing here the example of Thunder Client
but you can do the same thing using Postman
POST
request. Set Accept
Authorization
& X-GitHub-Api-Version
parameter
inside the body
as JSON Content
, in our case just the branch
name will be enough