Understanding Side Effects in Reactjs
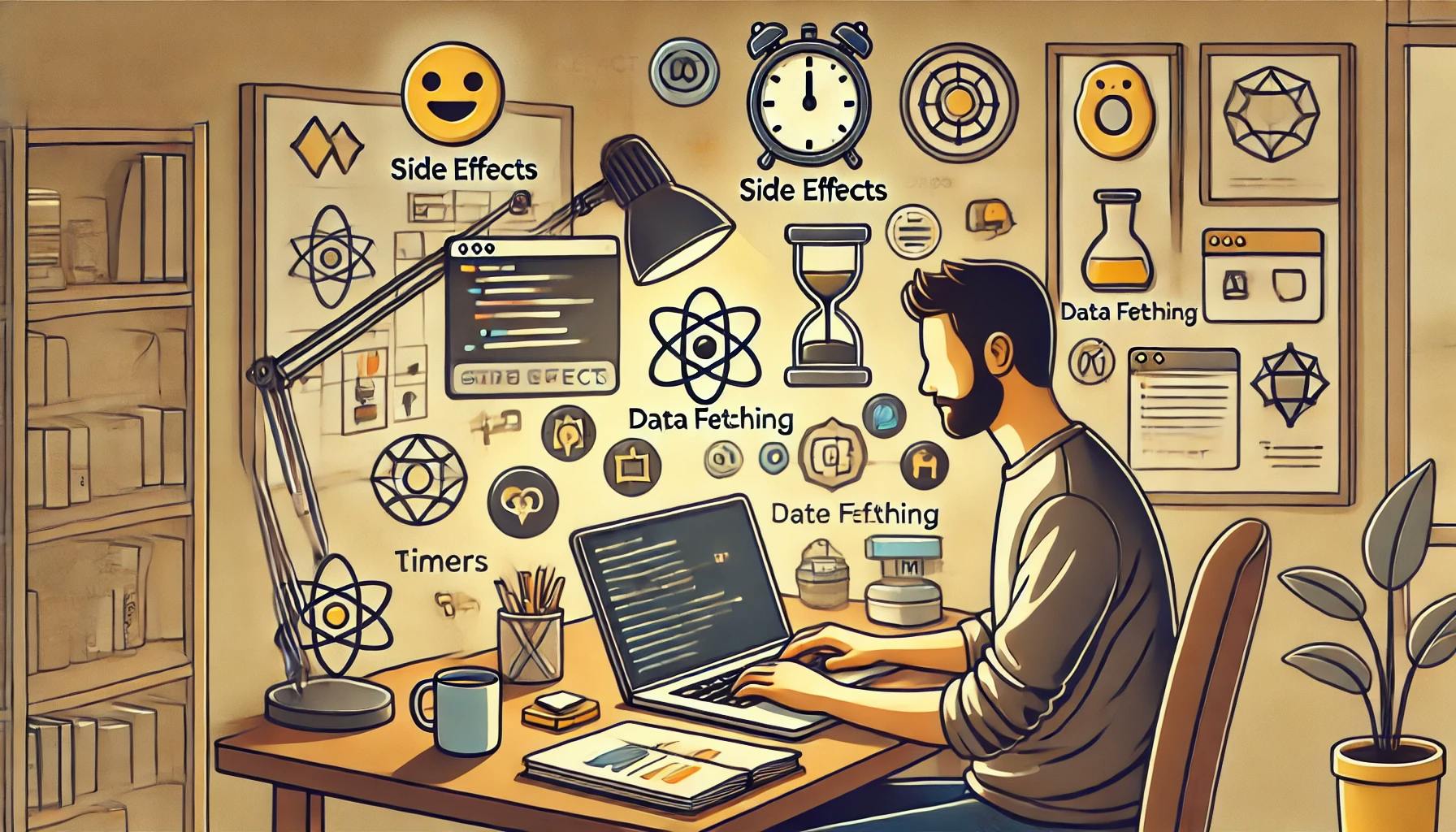
A side effect in React refers to any operation that interacts with the world outside React's component lifecycle. This means it's something that affects the browser, external APIs, or other external systems, rather than just updating the component's state or UI.
In React, the framework’s goal is to manage the user interface efficiently, while side effects often involve external interactions. For example, fetching data from an API or manipulating the DOM outside of React’s control introduces side effects into the application.
Here are some common examples of side effects in React applications:
document.getElementById
or querySelector
bypasses React’s virtual DOM, making it a side effect. setTimeout
or setInterval
interact with the browser’s execution flow, making them examples of side effects. Side effects are crucial for building functional applications, but they must be managed properly to prevent issues like memory leaks or race conditions.
React is a declarative framework, where developers describe the desired state of the UI, and React efficiently handles updates to the DOM. In contrast, side effects interact with systems outside React’s control, such as APIs or browsers. This separation ensures React can remain focused on rendering the UI without directly managing side effects.
Potential problems with side effects include:
To keep components predictable and easy to maintain, it's essential to manage side effects correctly.
React provides the useEffect
hook to handle side effects in a controlled manner. This hook allows developers to define what side effects to perform and when. For example, you can use useEffect
to:
The useEffect
hook ensures that side effects run at the right time (such as when a component mounts or updates), and it also provides a way to clean up resources when the component unmounts, preventing memory leaks.
By properly using the useEffect
hook, developers can write cleaner, more predictable, and easier-to-maintain React components.