How to Update Doctypes After Data Entry using Frappe patches
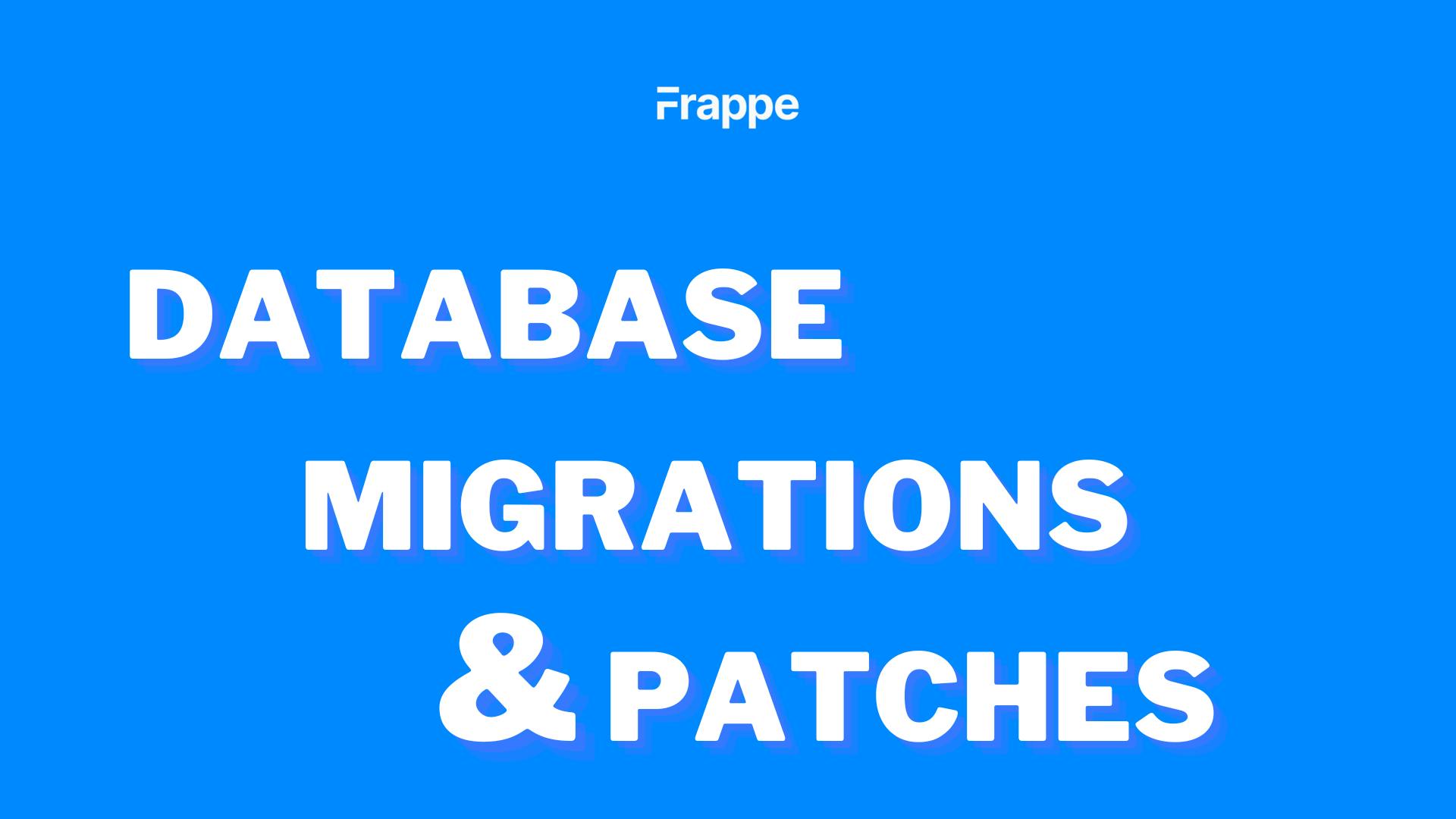
Patches are custom scripts that allow us to modify data
in Frappe
whenever we run the migrate
command.
The key feature of patches
is that they only run once, making them perfect for updating data
after making changes to your doctypes
or system configurations
.
Let’s explore this concept with a common scenario:
Imagine you have a doctype
with over 100
entries, and you've just made some changes to the doctype
structure.
Now, you need to update the content of all the existing records. Doing this manually would be time-consuming and prone to errors.
Luckily, we can use Frappe patches
to handle this task efficiently.
First, decide which doctype entries need to be updated. For this example, let's assume we're working with a Vehicle
doctype and we want to update each entry.
Within the specific doctype, create a folder named patches
.
__init__.py
(leave this empty). set_title.py
.
In the set_title.py
file, define a function called execute()
. This function will contain the logic to update all relevant entries.
Here’s a basic template for the patch function:
import frappe
def execute():
pass
patches.txt
FileTo ensure Frappe runs your patch, you need to register it in the patches.txt
file. There are two sections in this file:
In our case, we'll add the patch to the post_model_sync
section since we want it to run after the migration:
[post_model_sync]
# Patches added in this section will be executed after doctypes are migrated
rental.rental.doctype.vehicle.patches.set_title
Once the patch is ready, simply run the following command:
bench migrate
Frappe will automatically run your patch and update all the entries in the selected doctype.
Here’s a complete example of a patch that updates the title of each vehicle in the Vehicle
doctype:
import frappe
def execute():
# Get all vehicle entries
vehicles = frappe.db.get_all('Vehicle', pluck='name')
# Loop through each vehicle and update the title
for v in vehicles:
vehicle = frappe.get_doc('Vehicle', v)
vehicle.set_title()
vehicle.save()
# Commit the changes to the database
frappe.db.commit()
This patch retrieves all Vehicle
records, updates their title by calling the set_title()
method, and then saves the changes. Finally, the changes are committed to the database.