Understanding ORM A Beginner’s Guide to Database Management
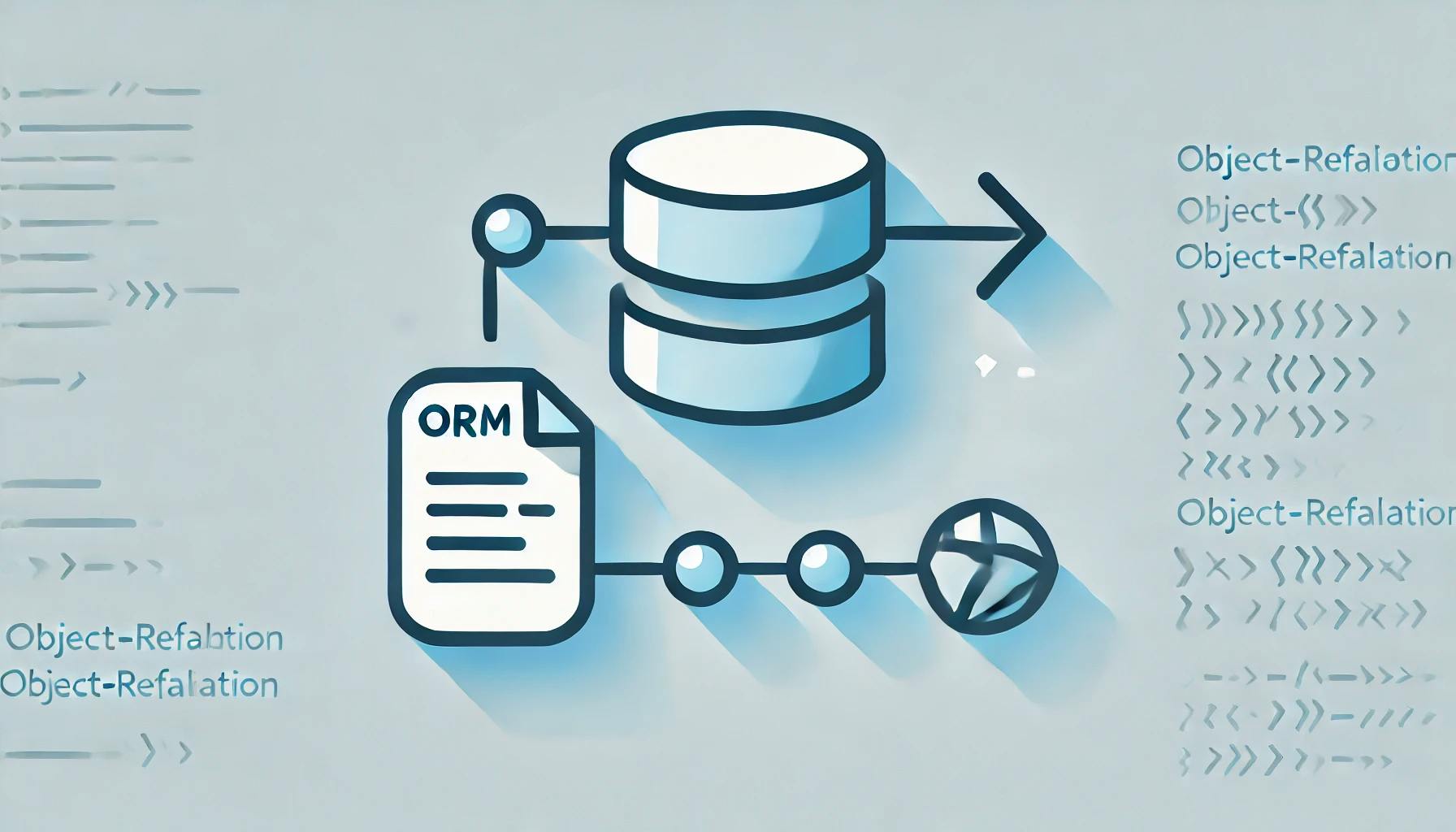
Object-Relational Mapping (ORM) is a way for programmers to interact with a database using simple code instead of writing complex database queries. ORM allows developers to work with data like it’s an object in their programming language, rather than writing SQL commands directly. This makes working with databases easier and less time-consuming.
Before ORM, developers had to write SQL queries manually to communicate with databases. This could be complicated and prone to mistakes. Around the early 2000s, ORM tools started to appear to make this process easier. Some of the first ORM tools included:
Feature | ORM | Manual Queries |
---|---|---|
Ease of Use | Uses simple code instead of SQL. | Requires knowledge of SQL syntax. |
Readability | Code is clean and easy to understand. | Queries can be long and complex. |
Performance | Might be slightly slower due to extra processing. | Usually faster because queries are written directly. |
Security | Protects against SQL injection automatically. | Developers must manually handle security risks. |
Compatibility | Works with different database types easily. | Queries may need to be rewritten for different databases. |
ORM is widely used in web development frameworks to simplify database management. Here’s how different frameworks use ORM:
Laravel has Eloquent, which makes database operations easy with simple code:
// Get a user by email
$user = User::where('email', 'example@example.com')->first();
// Create a new user
$user = new User();
$user->name = 'John Doe';
$user->email = 'john@example.com';
$user->save();
Frappe provides an ORM that helps in managing data within ERPNext applications:
# Get a user by email
user = frappe.get_doc("User", {"email": "example@example.com"})
# Create a new user
doc = frappe.get_doc({
"doctype": "User",
"email": "john@example.com",
"full_name": "John Doe"
})
doc.insert()
Django has a built-in ORM that allows working with databases using Python objects:
# Get a user by email
user = User.objects.get(email="example@example.com")
# Create a new user
user = User.objects.create(name="John Doe", email="john@example.com")
WordPress does not have a full ORM, but WPDB
helps interact with databases:
global $wpdb;
$user = $wpdb->get_row("SELECT * FROM wp_users WHERE user_email = 'example@example.com'");
Ruby on Rails uses Active Record for simple database operations:
user = User.find_by(email: "example@example.com")
user = User.create(name: "John Doe", email: "john@example.com")
NestJS uses TypeORM for database management:
const user = await userRepository.findOne({ where: { email: "example@example.com" } });
FastAPI works with SQLAlchemy to manage databases efficiently:
user = db.query(User).filter(User.email == "example@example.com").first()